.NET 7: What's new in ASP.NET Core?
Published: Tuesday 8 November 2022
.NET 7 has just been released with Visual Studio 2022, and we'll show you how to download and install it.
Afterwards, we'll look at some of the features, and what's new in ASP.NET Core.
Finally, we'll demonstrate the new features in action, and where to find a list of all the improvements for what promises to be the fastest .NET yet.
Install and download .NET 7
.NET 7 can be used with Visual Studio 2022 version 17.4 or above. The .NET 7 SDK may be installed with the Visual Studio installation. Otherwise, it can be downloaded from the Microsoft website. The SDK allows for building web apps using ASP.NET Core and comes with the ASP.NET Core Runtime. This is ideal for development.
Running an app on a server only requires the ASP.NET Core Runtime to be installed. Running ASP.NET Core apps on a Windows machine using IIS requires the ASP.NET Core Module V2 to be installed. Therefore, it's recommended to install the Windows Hosting Bundle.
Like with other recent updates of .NET, apps can be built and run-on Linux and Mac machines as well as Windows.
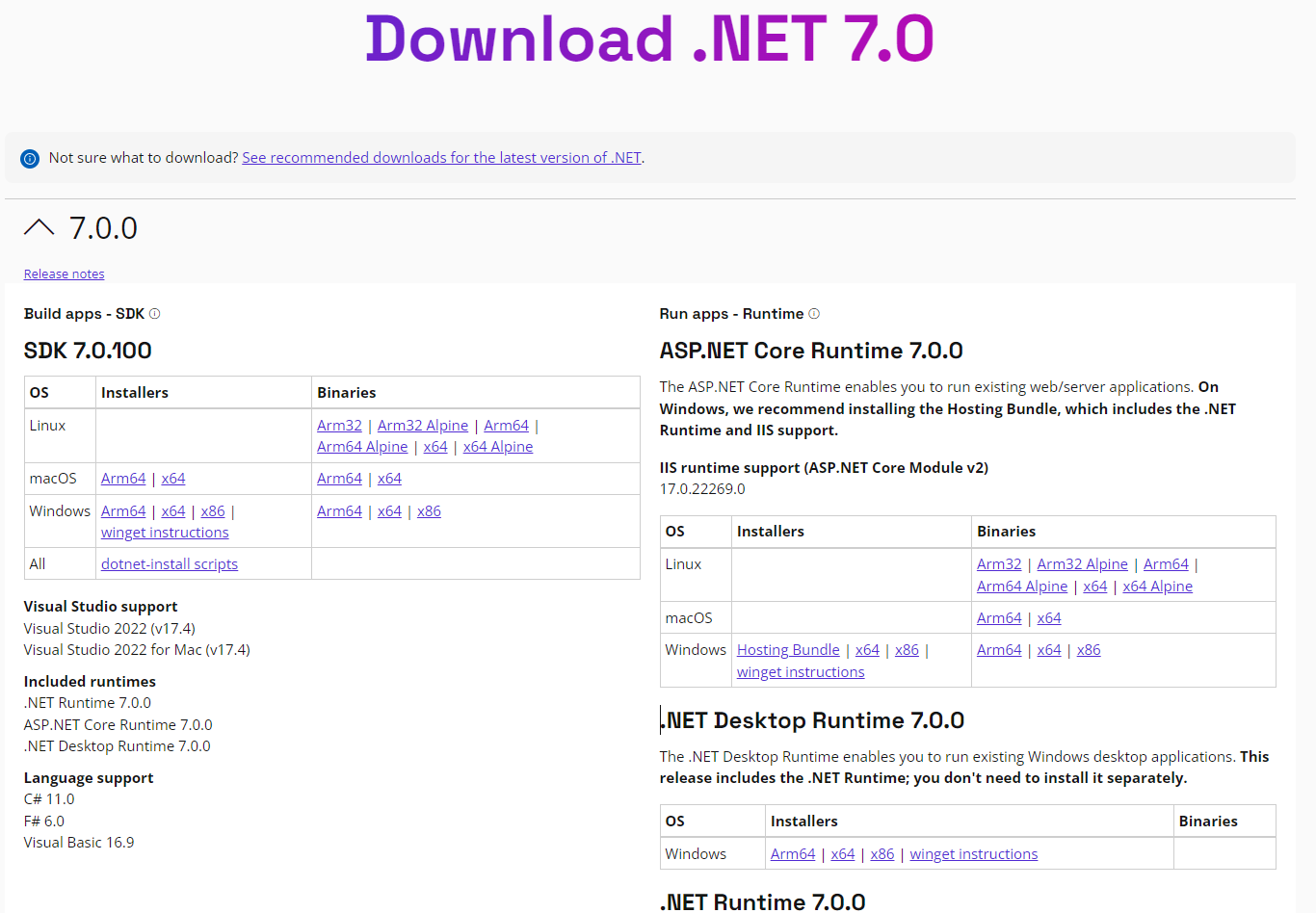
Download and install .NET 7 to use ASP.NET Core
New features in .NET 7
With .NET 7 installed, let's have a look at some of the new features that can be used with ASP.NET Core to build a web app.
Rate limiter
.NET 7 provides a rate limiter middleware for setting rate limiting policies. Web apps can configure these policies in Program.cs
and then use them with API endpoints.
There are a several different rate limiter options, including:
- Fixed window
- Sliding window
- Token bucket
- Concurrency
Looking at the fixed window option in more detail, it uses a fixed amount of time to limit requests. Once the time has elapsed, the request limit is reset.
In the example below, we've created a TestPolicy
fixed window limiter. The policy permits one request every 12 seconds.
We've also set a queue limit of 3, meaning that 3 requests will be left hanging until they can be processed. If more than 3 requests are queued, subsequent requests return a 503 Service Unavailable
error.
// Program.cs
...
builder.Services.AddRateLimiter(_ => _
.AddFixedWindowLimiter(policyName: "TestPolicy", options =>
{
options.PermitLimit = 1;
options.Window = TimeSpan.FromSeconds(12);
options.QueueProcessingOrder = QueueProcessingOrder.OldestFirst;
options.QueueLimit = 3;
}));
var app = builder.Build();
app.UseRateLimiter();
...
To use this policy with an API endpoint, the EnableRateLimiting
attribute can be assigned to it. It expects the policy name as a parameter of that attribute. As the policy is called TestPolicy
, that's the parameter that we will pass into the attribute.
// RateLimiterController.cs
[ApiController]
[Route("[controller]")]
public class RateLimiterController : ControllerBase
{
[EnableRateLimiting("TestPolicy")]
[HttpGet]
public IEnumerable<int> Get()
{
return new List<int>
{
{ 2 },
{ 4 },
{ 6 }
};
}
}
Parameter binding with dependency injection in API controllers
When returning an ActionResult
response in an API endpoint, we can now inject a type that is configured in dependency injection.
For this to work in .NET 6, we would have to use the [FromServices]
attribute:
public class MyController : ControllerBase
{
[HttpGet]
public ActionResult MyTime([FromServices] IMyService myService)
{
return Ok(new { Time = myService.MyTime });
}
}
With .NET 7, we can remove this attribute and it will still bind the service that is configured in dependency injection.
public class MyController : ControllerBase
{
[HttpGet]
public ActionResult MyTime(IMyService myService)
{
return Ok(new { Time = myService.MyTime });
}
}
OpenAPI improvements for Minimal APIs
.NET 7 sees the Microsoft.AspNetCore.OpenApi
package launched. This allows us to provide annotations to endpoints that are defined in Minimal APIs. Descriptions can be added for parameters, responses, and metadata.
In the example below, we've created a Minimal API GET endpoint with a route of /minimal-api
. It expects a count
parameter with a type of int
, where we've specifed a description for it:
// Program.cs
...
app.MapGet("/minimal-api", (int count) =>
{
return count;
})
.WithOpenApi(op =>
{
op.Parameters[0].Description = "Represents the count";
return op;
});
...
When running the application and opening the Swagger documentation, we can see that the description is added when examining that API endpoint:
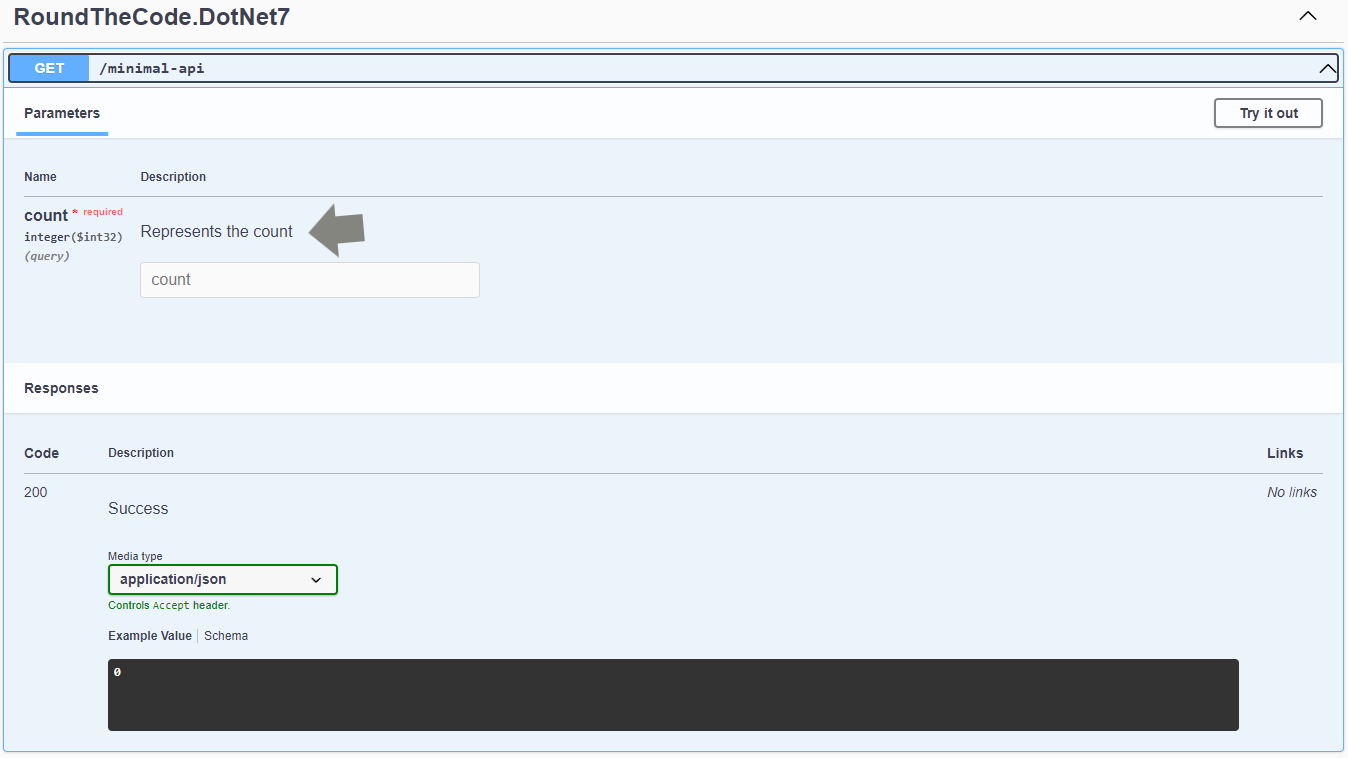
OpenAPI annotation with Minimal APIs in .NET 7
File uploads in Minimal APIs
Minimal APIs now support file uploads using the IFormFile
and IFormFileCollection
types.
The following example configures the Minimal API endpoint to upload a single file. The file is uploaded with the filename MyFile.jpg
, and that filename is returned as part of the response.
app.MapPost("/upload-file", async (IFormFile file) =>
{
var fileName = "MyFile.jpg";
using var stream = File.OpenWrite(fileName);
await file.CopyToAsync(stream);
return fileName;
});
More resources
Check out our video where we look at the new features covered in this article and demonstrate how they work.
In-addition, the new features included in the video are available to download so you can try out for yourself.
Finally, Microsoft have a full list of what's new in ASP.NET Core in .NET 7 where they detail all the new features, and give examples on how to use them.
Latest articles
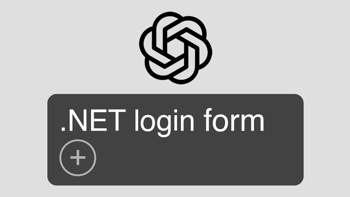
Can ChatGPT build an ASP.NET Core login form in 10 minutes?
We asked ChatGPT to write the code for a register and login form for an ASP.NET Core MVC application in 10 minutes and reviewed the code to see if it compiled.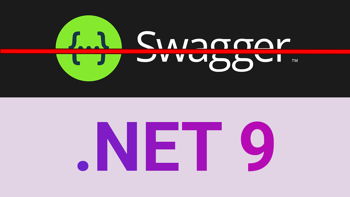