- Home
- .NET tutorials
- Allow your users to login to your ASP.NET Core app through Facebook
Allow your users to login to your ASP.NET Core app through Facebook
Published: Wednesday 23 September 2020
ASP.NET Core makes it easy to allow your users to login to your application through Facebook.
Facebook has billions of active users all over the world! With Facebook so popular, why wouldn't you integrate it?
Integrating Facebook has many benefits including:
- Being able to capture personal details from a users Facebook account, such as name and email
- Users not having to fill out another form to sign up for something
I personally like the fact that when you can log in through Facebook, it just makes the whole process quicker than usual.
Now that we've got reasons to integrate Facebook, we need to know how to do it.
The first thing you need to do is to set up an app in Facebook that users can connect to.
So, How Do I Setup an App in Facebook?
Well you need a Facebook account, but you probably already knew that.
You need to navigate to the Facebook for Developers website and click on the Log In link in the top-right corner of the screen.
nce logged in, or if you are already logged in, you need to click on either the Setup or My Apps link, again in the top-right corner of the screen.
If you don't already have a Facebook Developers account, you will need to go through the steps of setting one up. This will include either adding your mobile number, or a payment option, such as a credit card.
Once done, you will be redirected to a page that lists all your apps.
You will need to add a new app. When promoted how you are going to use your app, select "For Everything Else".
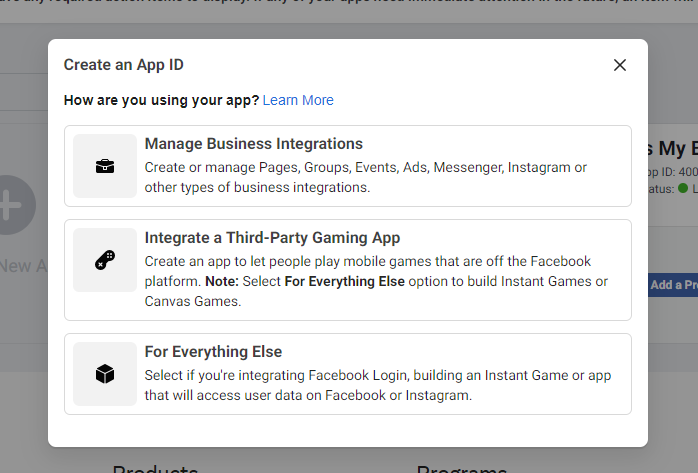
When creating an app in Facebook, select "For Everything Else"
You will then need to give it a Display Name and a Contact Email.
I Created The App. How Do I Integrate Facebook Login?
Now that you have created your app, you are greeted with a list of products that you can integrate with your Facebook app. You will need to set up the Facebook Login product.
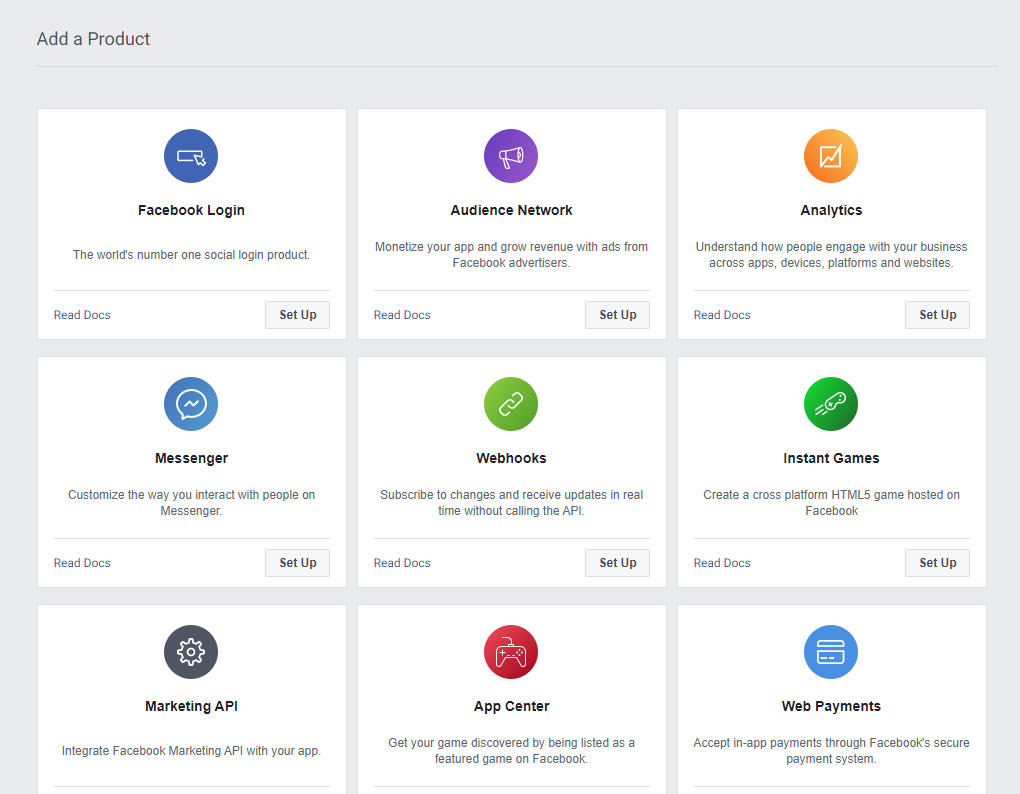
Set Up Facebook Login when you have created your Facebook app
At this point, a Facebook Login link will appear on the left-hand side menu. Go ahead and click on Settings.
You then need to go ahead and specify a valid OAuth Redirect URI. You will need to set this as https://{your_host}/signin-facebook. So if your application is hosted on localhost:8888, your redirect URI would need to be set as https://localhost:8888/signin-facebook.
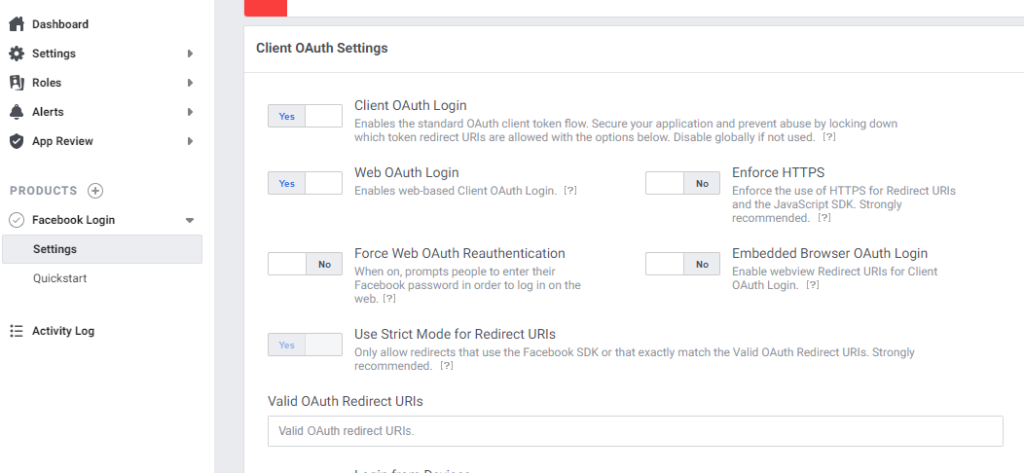
Click on Settings under Facebook Login on the left hand side. Then set the Valid OAuth Redirect URI with https://{your_host}/signin-facebook
Save the changes. Now you need to get your Facebook App Id & Secret.
On the left-hand side, go to Settings and Basic. You will need to retrieve your App Id & Secret.
The App Secret is hidden and you will need to reveal it. This may require you to re-login to your Facebook account.
Once you have it, make a note of both as we will need to add them in to our ASP.NET Core application later on.
How do I Integrate My Facebook App into my ASP.NET Core App?
I recommend setting up an ASP.NET Core MVC project in Visual Studio to follow this as we are going to use the default project that it creates for us.
The first thing we need to do is to add a Nuget package into our ASP.NET Core application. Open up Package Manager Console in Visual Studio and run the following command:
Install-Package Microsoft.AspNetCore.Authentication.Facebook
This package will contain all the functionality required to set up Facebook authentication.
Setting the App Id and Secret
We now need to configure our ASP.NET Core application to set up Facebook authentication. In-order to do this, we need to make some changes to our Startup class.
// Startup.cs
public class Startup
{
...
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthentication(options =>
{
options.DefaultScheme = CookieAuthenticationDefaults.AuthenticationScheme;
})
.AddCookie(options =>
{
options.LoginPath = "/account/facebook-login"; // Must be lowercase
})
.AddFacebook(options =>
{
options.AppId = "1066....";
options.AppSecret = "Bm....";
...
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
...
// Must be before UseEndPoints
app.UseAuthentication();
app.UseAuthorization();
...
}
}
The first thing we do is call AddAuthentication and set up a default scheme. We will use the CookieAuthenticationDefaults scheme as the default.
Afterwards, using the AddCookie method, we need to set up a default path for where a user is redirected when they require authentication. We have set this up as /account/facebook-login, and we will set this up later on in the tutorial.
Finally, using the AddFacebook method, we provide our app ID and app secret. This allows us to integrate our ASP.NET Core application with Facebook.
How to Require Authorisation to a Page?
We are going to require authorisation to the actions inside HomeController.cs. For that, we can use the [Authorize] attribute, which can be replaced as a class declaration. This means that all actions that sit in HomeController will require to be authorised.
However, we want to allow all traffic to our home page. To do that, we can add a [AllowAnonymous] attribute above our Index method.
/ HomeController.cs
[Authorize]
public class HomeController : Controller
{
...
[AllowAnonymous]
public IActionResult Index()
{
return View();
}
...
}
Now to Add the Login and Response Actions
We are going to create a new Account controller. This controller will contain two methods.
The first is a FacebookLogin method which will request authentication and send the user to a Facebook login screen. This will route to /account/facebook-login, which is what we set up as the LoginPath in our AddCookie method inside Startup.
The other is a Facebook Response method that will redirect the user back to the ASP.NET Core application once they've authenticated with our Facebook app. This is set up in our FacebookLogin method, using an instance of the AuthenticationProperties class.
When the FacebookResponse is called, we return the claims that Facebook have given us access to, such as a unique identifier, name and email.
// AccountController.cs
[AllowAnonymous, Route("account")]
public class AccountController : Controller
{
[Route("facebook-login")]
public IActionResult FacebookLogin()
{
var properties = new AuthenticationProperties { RedirectUri = Url.Action("FacebookResponse") };
return Challenge(properties, FacebookDefaults.AuthenticationScheme);
}
[Route("facebook-response")]
public async Task<IActionResult> FacebookResponse()
{
var result = await HttpContext.AuthenticateAsync(CookieAuthenticationDefaults.AuthenticationScheme);
var claims = result.Principal.Identities
.FirstOrDefault().Claims.Select(claim => new
{
claim.Issuer,
claim.OriginalIssuer,
claim.Type,
claim.Value
});
return Json(claims);
}
}
For your information, we've added an [AllowAnonymous] to the AccountController at class level. This is so you don't need authentication to view the methods in this controller.
I Still Can't This To Work
Watch our video coding tutorial.
We go ahead and create a Facebook app, setting up the app. With the app, we set up the Redirect URI and retrieve the app Id and secret.
Afterwards, we integrate Facebook authentication into an ASP.NET Core MVC app. We set up the Facebook Id and Secret. In addition, we set up actions where we redirect the user to the login back and display the data we get back from Facebook.
It's This Easy with Other Social Media Platforms
Regular readers to Round The Code may find that this is very similar to my article How to Add Google Authentication to a ASP.NET Core Application.
And that's because it is. The great thing about ASP.NET Core is that they have built in these authentications as part of ASP.NET Core.
All you need to do is to download a Nuget package from the Microsoft.AspNetCore assembly.
Now these tutorials use cookie authentication and a number of you may use a third-party solution, such as Identity Server.
You will need to check the instructions to see how exactly to integrate Facebook authentication with it. But you should be able to use the functionality from the Microsoft.AspNetCore.Authentication.Facebook to successfully log in your users to your application through Facebook.
And with it being this easy, why wouldn't you allow your users to login to your application via Facebook?
Related tutorials
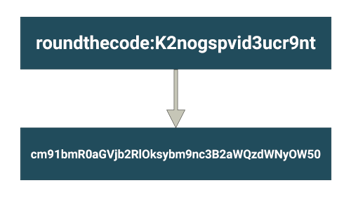
What is Basic authentication and how to add in ASP.NET Core
Learn what is Basic authentication and an example of how to add it to a HTTP request header in ASP.NET Core using Base64.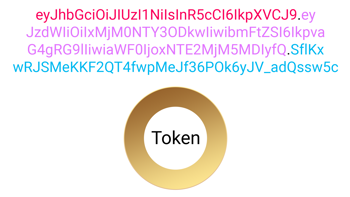
What is JWT and how to add it to ASP.NET Core
How to use JWT in ASP.NET Core for Bearer token authentication and security within the OAuth Client Credentials flow.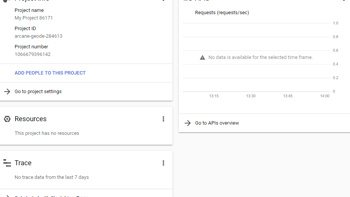