- Home
- .NET tutorials
- Server-side rendering (SSR) for Blazor in .NET 8
Server-side rendering (SSR) for Blazor in .NET 8
Published: Friday 20 October 2023
Server-side rendering (SSR) is a new Blazor feature for .NET 8.
It works in a similar way to ASP.NET Core MVC where the page is downloaded from the server and rendered to the browser.
We'll have a look at setting up a Visual Studio project, how it's configured in Program.cs
, how it loads into the browser and what happens when you try to delegate a button onclick
event.
Blazor Web App template in Visual Studio
A new Blazor Web App template has been created in Visual Studio for .NET 8 projects.
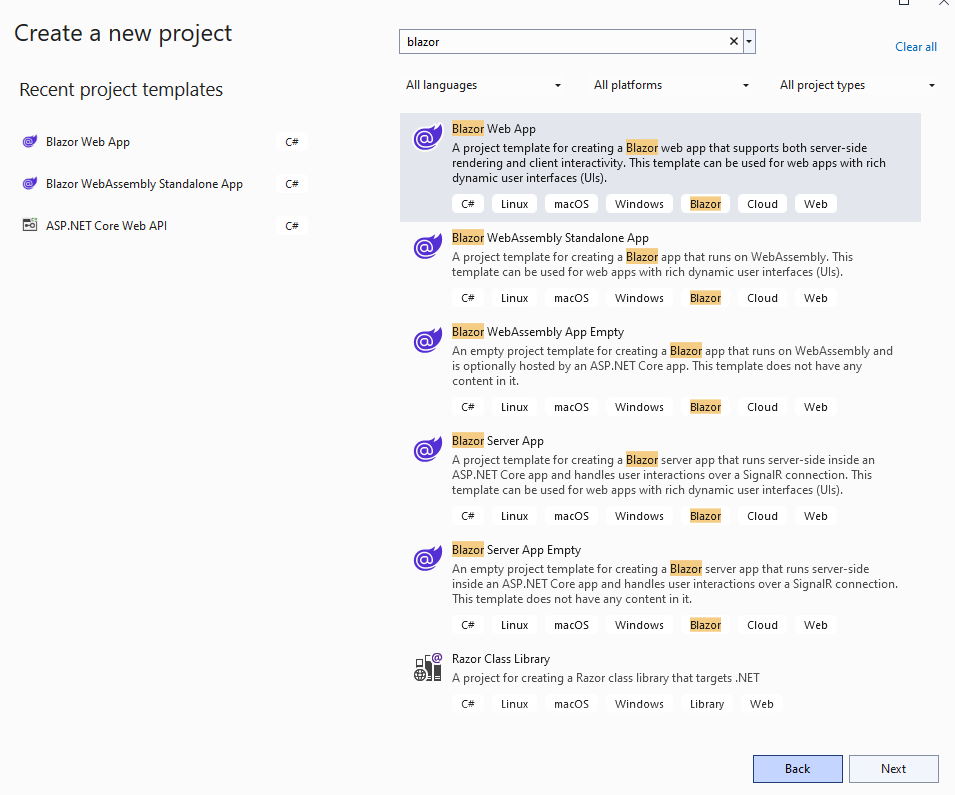
Blazor Web App project template in Visual Studio
Before setting up the project, it gives you a number of options that can be configured.
One of them includes the Interactivity type and this gives you the option to what render modes can be used for Razor components in the application.
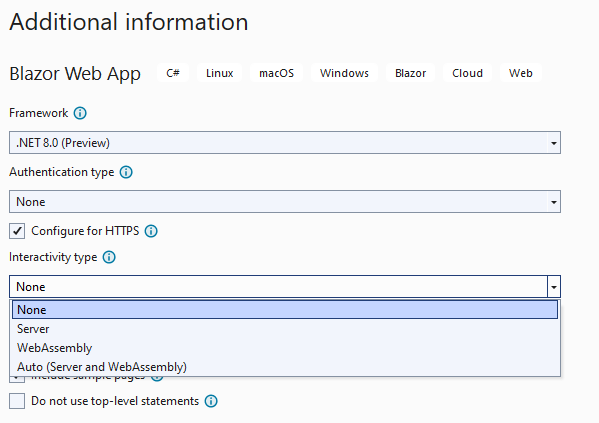
Blazor render modes for creating a project
If we wish to use SSR, we can select None from this dropdown. This will ensure that all components in our Blazor application will use the SSR render mode unless specified.
Project configuration
In Program.cs
, the AddRazorComponents
extension method is added to the IServiceCollection
instance. This registers the services required for SSR.
In-addition, the WebApplication
instance calls the MapRazorComponents
to a Razor component. By default, this goes to the App
component.
Here is an example of the code:
// Program.cs
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddRazorComponents();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Error", createScopeForErrors: true);
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseAntiforgery();
app.MapRazorComponents<App>();
app.Run();
How the application loads in the browser
The web browser downloads the component from the server and renders it. This is similar to ASP.NET Core MVC, but there is one major benefit to it.
When clicking between pages, it only does a partial update of the application and requests the resources from the server that it needs to update. As a result, any CSS/JavaScript files that were downloaded as part of the initial request aren't re-downloaded for each subsequent click.
This is different to MVC which refreshes the page, and re-downloads all the media for each click.
This image shows the web application loading on the Home page and then clicking on the Weather page. On the Weather page click, it only downloaded the weather content and updated the favicon
image.
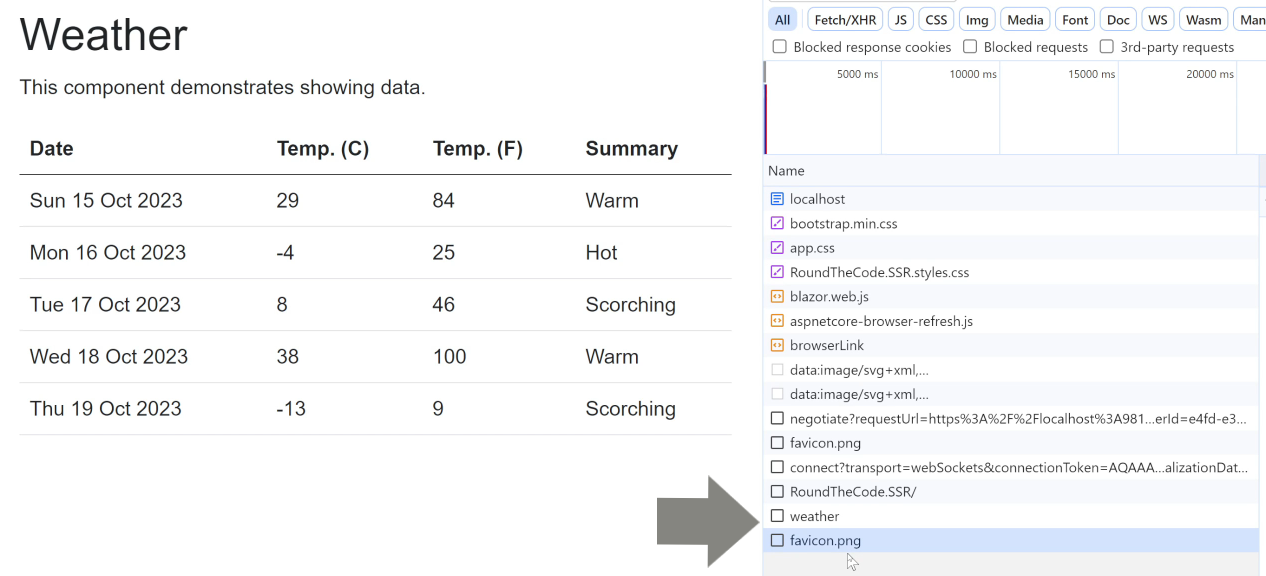
Blazor does a partial update with link clicks
Front-end functionality doesn't work
Unlike Server and WebAssembly render modes, front-end functionality doesn't work with SSR.
This can be tested by trying to change the text of an on-screen label when clicking on the button.
The button's onclick
attribute is rendered to the ClickMe
method and it should change the text from "Hello, world" to "Click me".
But when clicking on the button, nothing happens. The text doesn't update!
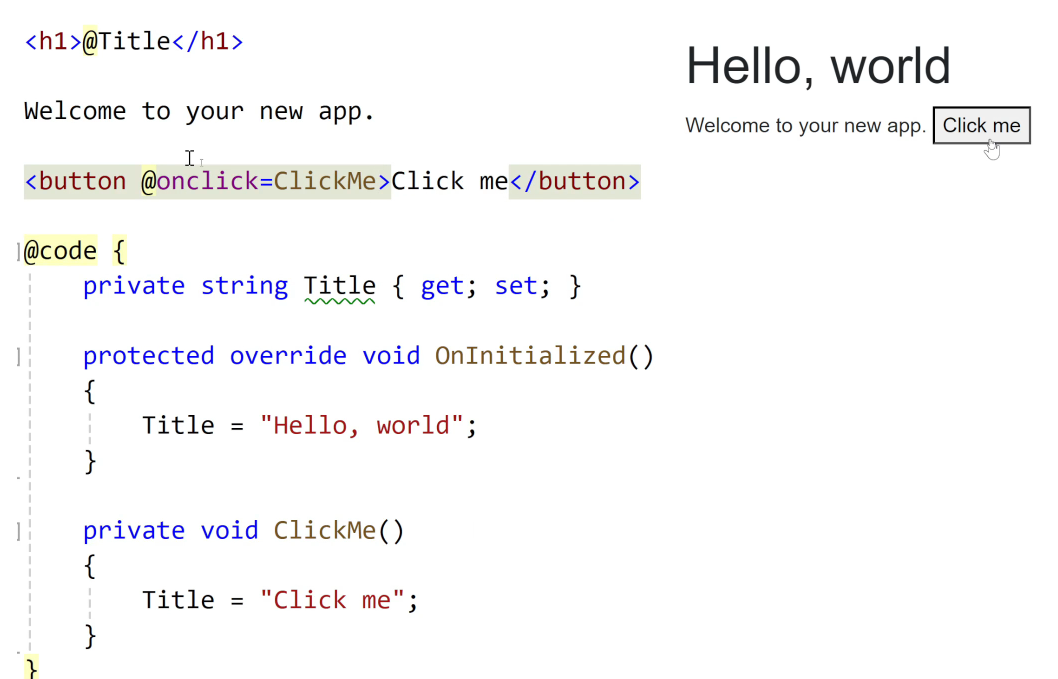
Button onclick doesn't work with Blazor SSR
If this interactivity is required within a component, then either the Server, or WebAssembly render mode will need to be chosen.
Watch the video
Watch our video where we set up a Blazor web app with SSR, go through the configuration, how it works in the web browser and what happens with a button onclick
event.
Related pages
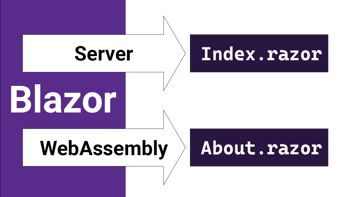
Render mode for Server & WebAssembly Blazor components
Blazor has a .NET 8 render mode feature that allows different modes to be set for Razor components in the same app whether it's Server, WebAssembly or Auto.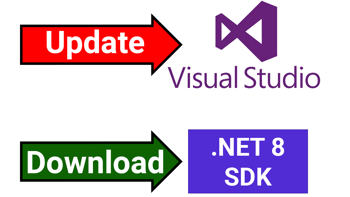