- Home
- .NET tutorials
- Data annotations has some awesome additions in .NET 8
Data annotations has some awesome additions in .NET 8
Published: Tuesday 5 December 2023
.NET 8 has brought some amazing additions to data annotations that allow for more flexibility when performing model validation in ASP.NET Core.
We'll have a look at each one in more detail and explain how each one works.
Specifying the allowed values
The AllowedValues
attribute allows for adding accepted values to a property. The values are an object
type meaning they can be specified with the property type. That means it could be used with a string
, int
or even anĀ enum
.
In this example, the Fruit
property in MyValidationModel
class has to be set to eitherĀ banana
or apple
for it to validate.
// MyValidationModel.cs
public class MyValidationModel
{
[AllowedValues("banana", "apple")]
public string Fruit { get; set; }
}
Denying certain values
The DeniedValues
attribute is the exact opposite to the AllowedValues
attribute which denies certain values to a property.
It will work with the type that is set in the property.
In this example, the Age
property can't be 16
or 17
otherwise it will not validate.
// MyValidationModel.cs
public class MyValidationModel
{
[DeniedValues(16, 17)]
public int Age { get; set; }
}
Validating a Base64 encoded string
The Base64String
attribute ensures that a string has to be Base64 encoded. This ensures that the property can only be validated if it has been encoded.
In this example, the Hash
property needs to be Base64 encoded otherwise a model validation error will occur.
// MyValidationModel.cs
public class MyValidationModel
{
[Base64String]
public string Hash { get; set; }
}
Specifying the length of a list type
If we have a List
type and we want to validate the minimum and maximum number of items, we can use the Length
attribute.
In this example, we wish to add our favourite fruits but we must specify between 1 and 3 items in the list for it to validate.
// MyValidationModel.cs
public class MyValidationModel
{
[Length(1, 3)]
public List<string> FavouriteFruits { get; set; }
}
Range has IsExclusive added
The Range
attribute has been around for a while, but there are additional optional parameters that have been added.
When either the MinimumIsExclusive
or MaximumIsExclusive
is set to true, it will exclude the minimum or maximum from the range and will not validate.
In this example, the range is set from 10 to 19. However, because both the MinimumIsExclusive
and MaximumIsExclusive
are set to true, it will throw a validation error if the age is either 10 or 19.
// MyValidationModel.cs
public class MyValidationModel
{
[Range(10, 19, MinimumIsExclusive = true, MaximumIsExclusive = true)]
public int Age { get; set; }
}
Web API demo
Watch our video where we demonstrate how to implement these new data annotations into an ASP.NET Core Web API controller endpoint and test the validation in Swagger to see if it works as we expect it to.
In-addition, download our code example which includes a model which is passed through an ASP.NET Core Web API controller and can be tested in Swagger.
Related pages
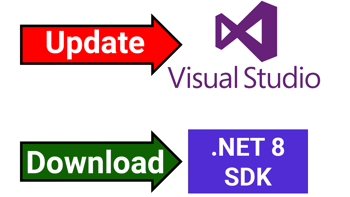
How to download and install the .NET 8 and C# 12 release
.NET 8 and C# 12 have been released and we'll show you how to update Visual Studio and download the .NET 8 SDK.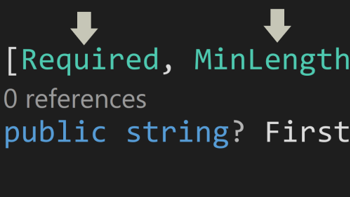