- Home
- .NET tutorials
- Collection expressions brings the spread operator to C# 12
Collection expressions brings the spread operator to C# 12
Published: Friday 3 November 2023
Collection expressions (also known as collection literals) is a new C# 12 feature that brings the spread operator to C#. This allows for common collection types, such as arrays, spans and lists to be joined into one.
In-addition, there is a syntax change for collection types which simplifies the code and helps to reduce code bloat.
Initialising a collection type
In versions prior to C# 12, initalising a collection type was quite bloated as we would have to specify the type that we are initalising.
Here is an example of how to initialise an array, span and a list with integers.
var a = new int[] { 1, 2, 3 };
var b = new Span<int>(new int[] { 2, 4, 5, 4, 4 });
var c = new List<int> { 4, 6, 6, 5 };
New syntax for C# 12
Going forward, we can use collection expressions to simplify the syntax of initalising a collection type.
We declare the variable with the collection type we want to use and then wrap the values around in square brackets.
int[] a =[1, 2, 3];
Span<int> b = [2, 4, 5, 4, 4];
List<int> c = [4, 6, 6, 5];
As we can see, it reduces a fair amount of code and looks easier to read.
The spread operator
The spread operator has been popular in JavaScript for joining and adding values to existing collection types.
And it finally makes its appearence in C#.
The spread operator can be used in collection types like arrays and inline arrays, as well spans and readonly spans. It can also be used in lists as well.
To use it, initalise a new collection type and then add ..
before adding the collection type variable.
int[] join = [..a, ..b, ..c];
With this type, additional values can also be added so if we wanted to add two additional values to the join collection type, we can do like this:
int[] join = [..a, ..b, ..c, 6, 5];
Changing collection types
It doesn't matter which collection types we are joining together, nor does it matter which collection type is being used for the variable that has the spread operator.
As long as it's an array, span, read only span or a list, we are able to use the spread operator to join collection types together, and declare that variable with any of those collection types.
int[] a =[1, 2, 3];
Span<int> b = [2, 4, 5, 4, 4];
List<int> c = [4, 6, 6, 5];
List<int> join = [..a, ..b, ..c, 6, 5];
Watch the video
Watch our video where we look at collection expressions in more detail. We show you how to use the spread operator to join collection types together, as well as the syntax change.
Related pages
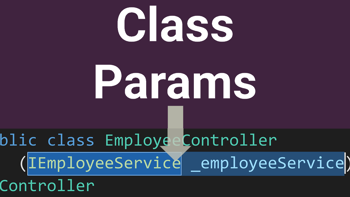
Primary constructors adds class parameters in C# 12
Primary constructors is a C# 12 feature that allows to add parameters to a class and includes dependency injection support.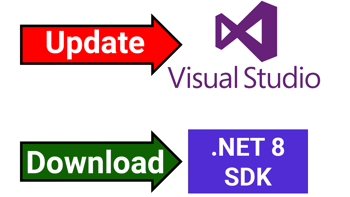