- Home
- .NET coding challenges
- Guess the SOLID principle from C# code - Part 2
Guess the SOLID principle from C# code - Part 2
The SOLID principles are important for the architecture of a .NET application as it can help reduce dependencies on other services.
SOLID is an acronym with each letter representing a principle:
- Single-responsibility principle
- Open–closed principle
- Liskov substitution principle
- Interface segregation principle
- Dependency inversion principle
Watch our video to find out more about them:
Take a look at this code:
public interface ICategory {
int Id { get; set; }
string? Name { get; set; }
}
public interface IProductCategory {
string? Description { get; set; }
}
public class BlogCategory : ICategory {
public int Id { get; set; }
public string? Name { get; set; }
}
public class ProductCategory : ICategory, IProductCategory {
public int Id { get; set; }
public string? Name { get; set; }
public string? Description { get; set; }
}
ICategory
and IProductCategory
have been created as interfaces.
In-addition, there is a BlogCategory
class that inherits the ICategory
interface. Finally, there is a ProductCategory
class that inherits both the ICategory
and IProductCategory
interfaces.
Which SOLID principle do you think we are following with this code?
Give us your anonymous feedback regarding this page, or any other areas of the website.
Related challenges
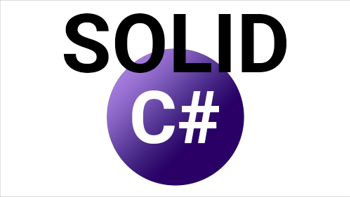