- Home
- .NET coding challenges
- Guess the SOLID principle from C# code
Guess the SOLID principle from C# code
The SOLID principles are important to follow when designing a .NET application as it can help reduce dependencies where one area can be changed without affecting others.
Each letter of the SOLID acronym represents one of the five principles. These principles are:
- Single-responsibility principle
- Open–closed principle
- Liskov substitution principle
- Interface segregation principle
- Dependency inversion principle
You can find out more about them by watching our video:
Look at this code:
public abstract class Vehicle
{
public abstract int Wheels { get; }
}
public class Car : Vehicle
{
public override int Wheels { get => 4; }
}
public class Motorbike : Vehicle
{
public override int Wheels { get => 2; }
}
public class Wheel {
public int CountTotalWheels(Vehicle[] vehicles) {
var wheelCount = 0;
foreach (var vehicle in vehicles) {
wheelCount += vehicle.Wheels;
}
return wheelCount;
}
}
We have created a Vehicle
abstract class which the Car
and Motorbike
classes inherit. The Vehicle class has an abstract Wheels
property which is overridden in both the Car
and Motorbike
class.
In-addition, we have a Wheel
class that has a CountTotalWheels
method. This passes an array of Vehicle
type instances and counts the number of the wheels.
Given the design of the code, which SOLID principle do you think we are following?
Related challenges
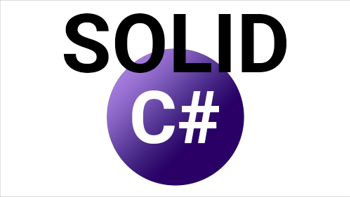