- Home
- .NET coding challenges
- Write a C# function to convert MPH to KPH
Write a C# function to convert MPH to KPH
If you have an application that involves recording the speed, you may need to convert miles-per-hour (MPH) to kilometers-per-hour (KPH).
Here is your chance to write a C# static function that allows you to convert MPH to KPH.
Start off with this code:
public class SpeedHelper
{
public decimal ConvertToKph(decimal mph)
{
}
}
Inside the SpeedHelper
class, there is a ConvertToKph
function. It expects an mph
parameter which is the speed in MPH which we wish to convert to KPH.
1 mile converts to 1.6093 km. Finish the ConvertToKph
function so it returns the value in KPH.
For example, if we passed in ConvertToKph(100)
, we would expect the return value to be 160.93 (100 * 1.6093).
Related challenges
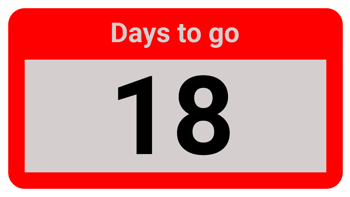
Work out the number of days between two dates
Write a C# function to work out the difference between two dates and return the number of days in this coding challenge.
Contains online code editor
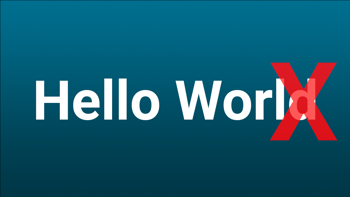
Removing the last character from a string
This coding challenge involves writing a C# method that will removing the last character from a string and returning the value.
Contains online code editor