- Home
- .NET coding challenges
- Using the yield statement in C#
Using the yield statement in C#
Yield in C# is an underused statement which can be used in an iterator to provide the next value or to signal the end of an iteration.
It can be used on collection types like a list or an array.
Microsoft provide a useful guide on how the yield statement works.
Take this code:
var oddNumbers = GetOddNumbers(new int[] { 1, 2, 3, 4, 5 });
foreach (var oddNumber in oddNumbers)
{
Console.WriteLine(oddNumber);
}
IEnumerable<int> GetOddNumbers(int[] numbers)
{
var oddNumbers = new List<int>();
foreach (int number in numbers)
{
if (number %2 == 1)
{
oddNumbers.Add(number);
}
}
return oddNumbers;
}
The output of this in a Console application would be:
1
3
5
How could we replace the functionality in the GetOddNumbers
method to include the yield
statement?
Related challenges
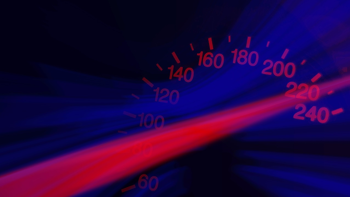
Write a C# function to convert MPH to KPH
Take our coding challenge to write a C# function with the necessary parameters that converts miles-per-hour (MPH) to kilometres-per-hour (KPH) and vice-versa.
Contains online code editor
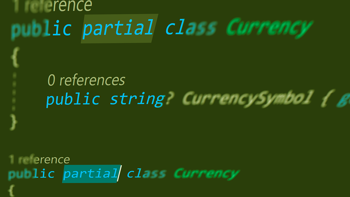