- Home
- .NET coding challenges
- How to read an appsettings.json value in ASP.NET Core
How to read an appsettings.json value in ASP.NET Core
In an ASP.NET Core Web API, you can inject the IConfiguration
instance as part of dependency injection.
Watch our video where we talk about how to read and get a value in ASP.NET Core:
We have this appsettings.json
file:
{
"Url": "https://www.roundthecode.com",
"Site": {
"Title": "Round The Code",
"Description": "ASP.NET Core tutorials"
},
"Smtp": {
"Port": 25
}
}
And we have this Web API controller that reads the configuration through IConfiguration
and returns the Url
key as part of the response for the SiteDetails
endpoint.
[ApiController]
[Route("api/site")]
public class SiteController : Controller {
private readonly IConfiguration _configuration;
public SiteController(IConfiguration configuration) {
_configuration = configuration;
}
[HttpGet]
public IActionResult SiteDetails() {
return Ok(new {
Url = _configuration.GetValue<string>("Url")
});
}
}
If we want to add Site:Title
and Smtp:Port
from appsettings.json
to the SiteDetails
endpoint response with a key of SiteTitle
and SmtpPort
respectively, how would we do it?
Related challenges
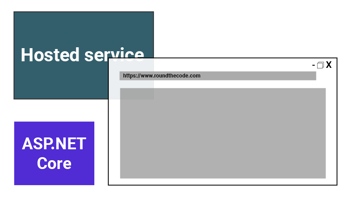
Add a hosted service to an ASP.NET Core app
See if you can add a background hosted service in an ASP.NET Core app with our .NET coding challenge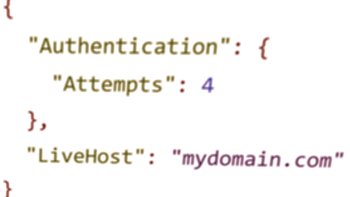