- Home
- .NET coding challenges
- Dependency injection circular dependency dangers in .NET
Dependency injection circular dependency dangers in .NET
Dependency injection is a popular design pattern used in ASP.NET Core for achieving Inversion of Control (or IoC) between classes and their dependencies.
Watch our video to learn more about dependency injection.
If the code architecture is poorly designed and services depend on one another, circular dependency can become an issue.
Take this code:
public interface ICategoryService { }
public class CategoryService : ICategoryService {
public CategoryService(IProductService productService) { }
}
public interface IProductService { }
public class ProductService : IProductService {
public ProductService(ICategoryService categoryService) { }
}
public interface ICartService { }
public class CartService : ICartService {
public CartService(IProductService productService) { }
}
If the ICategoryService
, IProductService
and ICartService
were all added as a service as part of dependency injection in ASP.NET Core, we would get a runtime error like this:
A circular dependency was detected for the service of type '{service}'.
Why is this?
Give us your anonymous feedback regarding this page, or any other areas of the website.
Related challenges
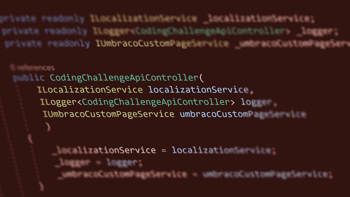
ASP.NET Core dependency injection service lifetimes
Learn about ASP.NET Core dependency injection and their service lifetimes with our C# coding challenge.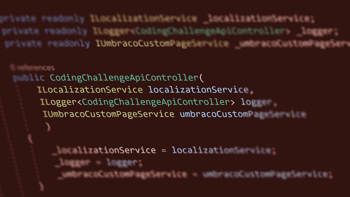