- Home
- .NET coding challenges
- Add services to dependency injection in .NET
Add services to dependency injection in .NET
With ASP.NET Core's dependency injection, you can add singleton, scoped and transient service lifetimes.
You can find out more about how dependency injection works in ASP.NET Core by watching our video.
We have three services that we wish to add to the IoC container in our ASP.NET Core web app.
The MySingletonService
inherits the IMySingletonService
. When adding this to the IoC container, we want to add it with a singleton service lifetime. As well as that, we wish to add the interface as the service and the class as the implementation.
public class MySingletonService : IMySingletonService {
}
public interface IMySingletonService {
}
The MyScopedService
inherits the IMyScopedService
. When adding this to the IoC container, we want to add it with a scoped service lifetime. As well as that, we wish to add the interface as the service and the class as the implementation.
public class MyScopedService : IMyScopedService {
}
public interface IMyScopedService {
}
The MyTransientService
inherits the IMyTransientService
. When adding this to the IoC container, we want to add it with a transient service lifetime. As well as that, we wish to add the interface as the service and the class as the implementation.
public class MyTransientService : IMyTransientService {
}
public interface IMyTransientService {
}
Here is an example of the Program.cs
that configures an ASP.NET Core app.
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
How would you add each service with the correct lifetime to the builder
instance?
Related challenges
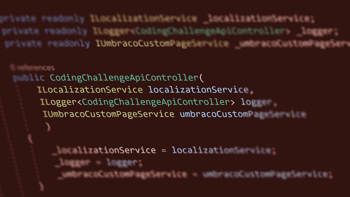
ASP.NET Core dependency injection service lifetimes
Learn about ASP.NET Core dependency injection and their service lifetimes with our C# coding challenge.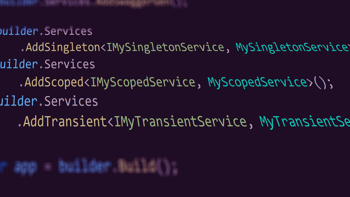